An Introduction to PowerFlex REST API Operations shows how to start using the powerful API of the PowerFlex solution.
We have written some PowerFlex articles. Click here to see them all!
In this article, I will focus only on PowerFlex API Operations. To learn more about PowerFlex, first, check out our other PowerFlex articles.
We are using PowerFlex version 4.5.2. So, our commands shown here were tested on this version!
We use the “curl” application to execute each API call.
First and foremost, what is an API?
An API (Application Programming Interface) is a set of rules and protocols that allows different software applications to communicate with each other. It defines the methods and data formats that applications can use to request and exchange information. APIs enable developers to access certain functionalities or data from an application, service, or system without understanding its internal workings.
For example, when you use a weather app, it may use an API to get weather data from a server (more than one in some situations). The API specifies how the app can request the weather data, what parameters it can send, and how the server will respond.
Do I need to install the PowerFlex API?
The PowerFlex API is installed as part of the PowerFlex Installation Manager/PowerFlex Gateway package.
The REST API is served from the PowerFlex Gateway through the REST gateway. The PowerFlex Gateway connects to a single MDM (metadata manager) and serves requests by querying the MDM and reformatting the answers it receives from the MDM in a RESTful manner back to a REST client. Every PowerFlex SCLI command is also available in the PowerFlex REST API. The responses returned by the PowerFlex Gateway are in JSON format.
PowerFlex API Operations
PowerFlex API operations are divided into three categories:
- The legacy API, which is called the block API. It is used to complete tasks related to block, protection, and operations on the core PowerFlex software.
- The PowerFlex Manager API manages the life cycle of PowerFlex rack and appliance deployments and core software.
- The PowerAPI, introduced in PowerFlex v4.0, manages SSO, NAS, files, events, and alerts. It is based on a new Dell Technologies standard for product APIs.
Eventually, the block API and PowerFlex Manager API will be deprecated, and their functions will be merged into the PowerAPI model. The web service for REST API is hosted on the PowerFlex management platform (PFMP), simplifying programmatic operations, including authentication.
PFMP uses the industry-standard OAuth 2.0 protocol for authorization. In OAuth 2.0, a user authenticates with an authorization microservice in PFMP. Upon authentication, the user is passed an access (bearer) token. The bearer token enables the user to access protected resources and perform protected operations on all areas of the PowerFlex system.
To read more about PowerFlex Block API:
https://infohub.delltechnologies.com/en-us/l/powerflex-rest-api-introduction/powerflex-block-api/
To read more about PowerFlex Manager API:
https://infohub.delltechnologies.com/en-us/l/powerflex-rest-api-introduction/powerflex-manager-api/
To read more about PowerFlex PowerAPI:
https://infohub.delltechnologies.com/en-us/l/powerflex-rest-api-introduction/powerflex-powerapi/
To get all details about all available APIs, check the following link:
https://developer.dell.com/apis/4008/versions/4.5.2/docs/Introduction/Introduction_powerAPI.md
First Things First: Acquiring the Authentication Token
Firstly, we need to acquire the authentication token. The following command can do it:
read -s -p "Enter Password: " PASSWORD &&
pfmp_token=$(curl -s -k --location --request POST 'https://pfmp.lab.local/rest/auth/login' \
--header 'Accept: application/json' \
--header 'Content-Type: application/json' \
--data-raw "{
\"username\": \"admin\",
\"password\": \"$PASSWORD\"
}" |jq -r '.access_token') && unset PASSWORD
Replace the “pfmp.lab.local” with your PowerFlex Manager FQDN.
Command explanation:
- The first command line uses “read” to ask yourself about the password of the username admin and stores it in a variable “PASSWORD”;
- The second command line calls the PowerFlex Manager cluster, asking for the authentication token. Look at the “data-raw” section. The “admin” username is used, and its password is a variable, created by the first command line;
- The “unset PASSWORD” removes the variable PASSWORD. This variable stores the admin password in a clear-text mode. So, for security reasons, we removed the authentication token after acquiring it;
- The authentication token is placed at the variable “pfmp_token”.
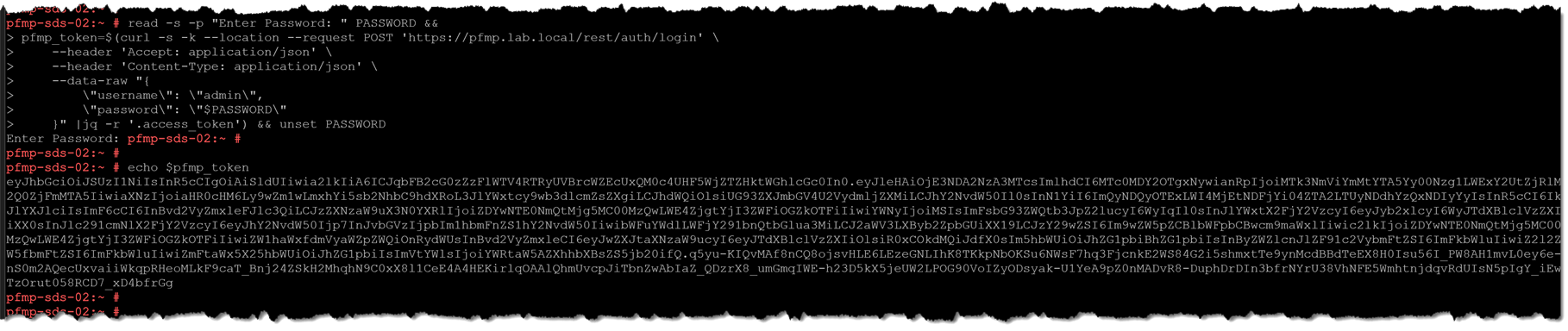
After acquiring the authentication token, we can perform API calls using it!
Important: An access token has an expiration date. The access token is valid only for 5 minutes. To continue accessing the system after the token expires, you can either update the token using standard OIDC refresh operation, or log in again to get a new access token.
If you try executing an API call and receiving an output like the following, it's probably because your authentication token expired:
pfmp-sds-02:~ # curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/System/instances
<html>
<head><title>401 Authorization Required</title></head>
<body>
<center><h1>401 Authorization Required</h1></center>
<hr><center>nginx</center>
</body>
</html>
System API Call Examples
# Grabbing the PowerFlex version:
curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/System/instances | jq -r '.[].systemVersionName'
# Grabbing the PowerFlex Installation ID:
curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/System/instances | jq -r '.[].installId'
# MDM cluster details:
curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/System/instances | jq -r '.[].mdmCluster'
# Grabbing the MDM cluster mode (1_node, 3_node, or 5_node):
curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/System/instances | jq -r '.[].mdmCluster.clusterMode'
# Grabbing the MDM Master/Primary node details:
curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/System/instances | jq -r '.[].mdmCluster.master'
curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/System/instances | jq -r '.[].mdmCluster.master.name'
# Grabbing the MDM Slave/Secondary nodes details:
curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/System/instances | jq -r '.[].mdmCluster.slaves'
curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/System/instances | jq -r '.[].mdmCluster.slaves[].name'
# Grabbing the MDM Tie-Breaker nodes details:
curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/System/instances | jq -r '.[].mdmCluster.tieBreakers'
curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/System/instances | jq -r '.[].mdmCluster.tieBreakers[].name'
SDC and SDS API Call Examples
# Grabbing all SDC details and their details like name, IP address, and MDM connection status:
curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/Sdc/instances | jq -r '.[].name'
curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/Sdc/instances | jq -r '.[] | "\(.name) - \(.sdcIp)"'
curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/Sdc/instances | jq -r '.[] | "\(.name) - \(.sdcIp) - \(.mdmConnectionState)"'
# Grabbing all SDS details and their details like name, Maintenance Mode status, and MDM connection status:
curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/Sds/instances | jq -r '.[] | "\(.name) - \(.maintenanceType) - \(.mdmConnectionState)"'
Protection Domain and Storage Pool API Call Examples
# Protection Domain:
# Grabbing all Protection Domains details:
curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/ProtectionDomain/instances | jq
# Name of all Protection Domains:
curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/ProtectionDomain/instances | jq -r '.[].name'
# Name and state of all Protection Domains:
curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/ProtectionDomain/instances | jq -r '.[] | "\(.name) - \(.protectionDomainState)"'
# Storage Pool:
# Grabbing all Storage Pools details:
curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/StoragePool/instances | jq
# Grabbing the name of all Storage Pools:
curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/StoragePool/instances | jq -r '.[].name'
# Grabbing the name, id, data layout (Medium or Fine Granularity), spare percentage, warning capacity threshold, and critical capacity threshold:
curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/StoragePool/instances | jq -r '.[] | "\(.name) - \(.id) - \(.dataLayout) - \(.sparePercentage) - \(.capacityAlertHighThreshold) - \(.capacityAlertCriticalThreshold)"'
Volumes API Call Examples
# Grabbing all PowerFlex Volumes details:
curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/Volume/instances
# Name of all PowerFlex Volumes:
curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/Volume/instances | jq -r '.[].name'
# Name and size (KB) of all PowerFlex Volumes:
curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/Volume/instances | jq -r '.[] | "\(.name) - \(.sizeInKb)"'
# Name, size (KB), access mode (Read/Write), and volume type (thin/thick provision) of all PowerFlex Volumes:
curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/Volume/instances | jq -r '.[] | "\(.name) - \(.sizeInKb) - \(.accessModeLimit) - \(.volumeType)"'
Creating a PowerFlex Volume Through API
All previous API calls are “GET” APIs, so they only retrieve values from the PowerFlex API gateway.
We will perform a new API call, a “POST” API:
1. Getting the Storage Pool ID:
curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/StoragePool/instances | jq -r '.[] | "\(.name) - \(.id) - \(.dataLayout) - \(.sparePercentage) - \(.capacityAlertHighThreshold) - \(.capacityAlertCriticalThreshold)"'
2. Creating the Volume:
curl -k -X POST https://pfmp.lab.local/api/types/Volume/instances \
-H "Content-Type: application/json" \
-H "Authorization: Bearer ${pfmp_token}" \
-d '{
"name": "Test_Volume_01",
"compressionMethod": "None",
"storagePoolId": "b0a820d300000000",
"volumeType": "ThinProvisioned",
"volumeSizeInGb": "32"
}'
Where:
Test_Volume_01 –> Volume name
b0a820d300000000 –> Storage Pool ID used to create the volume
ThinProvisioned –> Provision volume type
32 –> Volume size in GB
3. Checking if the Volume was created:
curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/Volume/instances | jq -r '.[].name' | grep -i Test_Volume_01
4. To map this Volume to an SDC host:
4.1. Getting the SDC ID:
curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/Sdc/instances | jq -r '.[] | "\(.name) - \(.id)"'
4.2. Mapping the Volume to an SDC through its SDC ID:
curl -k -X POST https://pfmp.lab.local/api/instances/Volume::0c1da31900000008/action/addMappedHost \
-H "Content-Type: application/json" \
-H "Authorization: Bearer ${pfmp_token}" \
-d '{
"sdcId": "1232221400000002",
"accessMode": "ReadWrite"
}'
4.3. Checking what SDC devices the volume has been mapped:
curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/Volume/instances | jq '.[] | select(.name == "Test_Volume_01") | {name, mappedSdcInfo}'
curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/Volume/instances | jq '.[] | select(.name == "Test_Volume_01") | {name, mappedSdcInfo: [.mappedSdcInfo[] | {sdcIp, sdcName, accessMode}]}'
Deleteting/Removing the PowerFlex Volume:
In this case, we will remove the volume created before. Before removing it, we removed all mapped SDC.
1. Getting the Volume ID to be removed:
curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/Volume/instances | jq -r '.[] | "\(.name) - \(.id)"'
2. Removing the Volume (replace the volume ID with your volume ID – be careful with that and check again if the volume ID is the correct one before removing it):
curl -k -X POST https://pfmp.lab.local/api/instances/Volume::0c1da31800000008/action/removeVolume \
-H "Content-Type: application/json" \
-H "Authorization: Bearer ${pfmp_token}" \
-d '{
"removeMode": "ONLY_ME"
}'
3. Check all Volumes and confirm if the Volume was removed:
curl -s -k -H "Authorization: Bearer ${pfmp_token}" https://pfmp.lab.local/api/types/Volume/instances | jq -r '.[] | "\(.name) - \(.id)"'
To Wrap This Up
Learning about PowerFlex APIs is crucial for effectively automating and streamlining tasks within a PowerFlex environment. By understanding how to interact with PowerFlex through its API, you can programmatically manage storage resources, monitor performance, and integrate PowerFlex with other systems or applications.
This knowledge enables more efficient administration, reduces manual intervention, and empowers you to build custom automation solutions that can improve scalability, reduce errors, and enhance overall operational efficiency. Mastering PowerFlex APIs also allows for deeper customization, helping you align storage operations with your organization’s specific needs and workflows.